In Java, we often create multiple classes, including functional classes such as service or utility classes that perform specific tasks. Additionally, we create classes solely for the purpose of storing or carrying data, a practice demonstrated by the use of record classes in Java 17.
For example:
public class Sample {
private final int id = 10;
private final String name = "Pavan";
}
Table of Contents
When to use Record Classes in Java
When our object is immutable and we don’t intend to change its data, we create such objects primarily for data storage. Let’s explore how to create such a class in Java.
class Student {
private final int id;
private final String name;
private final String college;
public Student(int id, String name, String college) {
this.id = id;
this.name = name;
this.college = college;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getCollege() {
return college;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", college='" + college + '\'' +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Student student = (Student) o;
return id == student.id && Objects.equals(name, student.name)
&& Objects.equals(college, student.college);
}
@Override
public int hashCode() {
return Objects.hash(id, name, college);
}
}
public class RecordTest {
public static void main(String[] args) {
Student s1 = new Student(1, "Pavan", "IIIT");
Student s2 = new Student(2, "Sachin", "Jntu");
Student s3 = new Student(2, "Sachin", "Jntu");
System.out.println(s1.getName());
System.out.println(s1);
System.out.println(s1.equals(s2));
System.out.println(s2.equals(s3)); //true
}
}
Ouput:
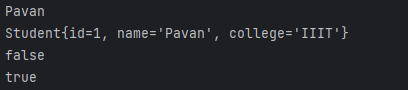
In this code, we’ve created a Student
class to represent student data, ensuring immutability by making fields id
, name
, and college
final. Additionally, we’ve overridden toString()
, equals()
, and hashCode()
methods for better readability and correct comparison of objects. Finally, we’ve tested the class in RecordTest
class by creating instances of Student
and performing some operations like printing details and checking for equality.
In Java 17, with the introduction of the records feature, the Student class can be replaced with a record class. It would look like this:
record Student (int id, String name, String college){}
public class RecordTest {
public static void main(String[] args) {
Student s1 = new Student(1, "Pavan", "IIIT");
Student s2 = new Student(2, "Sachin", "Jntu");
Student s3 = new Student(2, "Sachin", "Jntu");
//we don't have get method in records,
//we can acces name like below.
System.out.println(s1.name());
System.out.println(s1);
System.out.println(s1.equals(s2));
System.out.println(s2.equals(s3)); //true
}
}
Output:
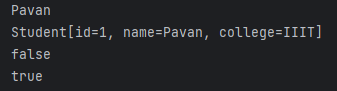
- Parameterized Constructors: Record classes internally define parameterized constructors. All variables within a record class are private and final by default, reflecting the immutable nature of records.
- Equals() Method Implementation: By default, a record class implements the equals() method, ensuring proper equality comparison between instances.
- Automatic toString() Method: The toString() method is automatically defined for record instances, facilitating better string representation.
- No Default Constructor: It’s important to note that record classes do not have a default constructor. Attempting to instantiate a record class without parameters, like
Student s = new Student();
, would result in an error. - Inheritance and Interfaces: Record classes cannot extend any other class because they implicitly extend the Record class. However, they can implement interfaces.
- Additional Methods: Methods can be added to record classes. Unlike traditional classes, record classes do not require getter and setter methods for accessing variables. Instead, variables are accessed using the syntax
objectName.varName()
. For example:s.name()
.
Java 21 Features with Examples
Latest Posts:
- React Server Side Rendering
- How Many Requests Can Spring Boot Handle Simultaneously?
- React Redux Essentials: A Comprehensive Guide
- Authentication and Authorization in React
- CRUD Operations in React: First CRUD Application
For additional information on Java 17 features, please visit