The Basics of JSX in React: Syntax, Expressions, and Examples
Introduction
In React, JSX (JavaScript XML) is a syntax extension that allows you to write HTML-like code directly in JavaScript files. JSX makes it easy to structure components in React, ensuring cleaner code and faster development. In this guide, we’ll explore JSX syntax, expressions, and examples, so you can effectively utilize JSX in your React applications.
Table of Contents
1. What is JSX?
JSX is a syntax extension to JavaScript that resembles HTML and allows us to write React elements directly. It’s transpiled by tools like Babel into standard JavaScript, which browsers understand.
JSX simplifies the way we write UI components by blending HTML with JavaScript logic, allowing developers to create dynamic, interactive web pages with less code.
2. Advantages of Using JSX in React
- Easier to Read and Write: JSX resembles HTML, making it more readable, especially for those familiar with web development.
- Powerful Integration with JavaScript: It enables the use of JavaScript within your UI components, making it easy to display dynamic content.
- Improves Performance: JSX helps React’s virtual DOM perform updates more efficiently.
3. JSX Syntax Basics
JSX syntax closely mirrors HTML with some essential differences and rules.
Basic Syntax
In JSX, elements are written similarly to HTML:
const element = <h1>Hello, JSX!</h1>;
Parent Elements
Every JSX expression must have a single parent element. If you want multiple sibling elements, wrap them in a <div>
or React Fragment (<> </>
).
const element = (
<div>
<h1>Hello, World!</h1>
<p>This is a paragraph in JSX.</p>
</div>
);
Self-Closing Tags
In JSX, elements without children must be self-closed (e.g., <img />
, <br />
).
const element = <img src="logo.png" alt="Logo" />;
4. Embedding Expressions in JSX
JSX allows you to embed any JavaScript expression by using curly braces {}
.
Example
const name = "React Developer";
const greeting = <h1>Hello, {name}!</h1>;
Conditional Rendering
JavaScript expressions also allow conditional rendering in JSX:
const isLoggedIn = true;
const userGreeting = (
<div>
<h1>Welcome {isLoggedIn ? "back" : "guest"}!</h1>
</div>
);
5. Practical Example
Example 1: Displaying an Array of Data in JSX
Let’s render a list of items dynamically:
const fruits = ["Apple", "Banana", "Cherry"];
const fruitList = (
<ul>
{fruits.map(fruit => (
<li key={fruit}>{fruit}</li>
))}
</ul>
);
Explanation: This code maps over an array, creating an
<li>
element for each item in thefruits
array. Each item requires a uniquekey
attribute for optimal rendering.
Example 2: Handling Events in JSX
JSX allows attaching event listeners directly:
function handleClick() {
alert("Button clicked!");
}
const button = <button onClick={handleClick}>Click Me!</button>;
Explanation: Here,
onClick
is bound to thehandleClick
function, which triggers an alert when the button is clicked.
6. Best Practices for Writing JSX
- Use Descriptive Variable Names: When naming JSX elements, ensure variables are descriptive and contextually relevant. It enhances readability.
- Break Down Complex Components: Large JSX blocks should be split into smaller components for easier testing and reuse.
- Use Keys When Rendering Lists: Always use unique keys when iterating over arrays to ensure React efficiently manages DOM updates.
- Avoid Inline Functions in JSX: Using inline functions can create performance issues as a new function is created every render. Define functions separately when possible.
// Less Optimal
const button = <button onClick={() => console.log("Clicked")}>Click Me!</button>;
// Optimal
function handleClick() {
console.log("Clicked");
}
const button = <button onClick={handleClick}>Click Me!</button>;
- Use Fragments Instead of Extra
<div>
Elements: React Fragments (<> </>
) avoid unnecessary HTML elements when returning multiple elements in JSX.
Example 3: Building a Dynamic To-Do List with JSX
In this example, we’ll use JSX to create a to-do list where users can add items dynamically. This will demonstrate how JSX handles user interactions, state, and renders lists in a React component.
Step 1: Setting Up the Component
First, create a new React component called TodoList
. We’ll use React’s useState
hook to manage our list of to-do items and the input text for new items.
import React, { useState } from 'react';
function TodoList() {
const [items, setItems] = useState([]); // State for the list of items
const [newItem, setNewItem] = useState(''); // State for the input text
const handleAddItem = () => {
if (newItem.trim() !== '') {
setItems([...items, newItem]); // Adds new item to the list
setNewItem(''); // Resets the input field
}
};
return (
<div>
<h2>My To-Do List</h2>
<input
type="text"
placeholder="Add new item"
value={newItem}
onChange={(e) => setNewItem(e.target.value)} // Updates input state on change
/>
<button onClick={handleAddItem}>Add Item</button>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li> // Renders each item with a unique key
))}
</ul>
</div>
);
}
export default TodoList;
Explanation of the Code
- State Management: We use
useState
to create two pieces of state:items
for the to-do list items.newItem
for the text currently in the input field.
- Event Handling:
handleAddItem
function adds the item to theitems
array and clears the input after adding.- The input field uses
onChange
to update thenewItem
state whenever the user types something.
- Rendering the List:
- The
items
array is mapped to an unordered list (<ul>
), where each item appears as a list item (<li>
). We use thekey
attribute to uniquely identify each item.
- The
Final Output
This component will display an input field, a button to add items, and a list of items below. Users can type an item, click “Add Item,” and see their items appended to the list in real-time.
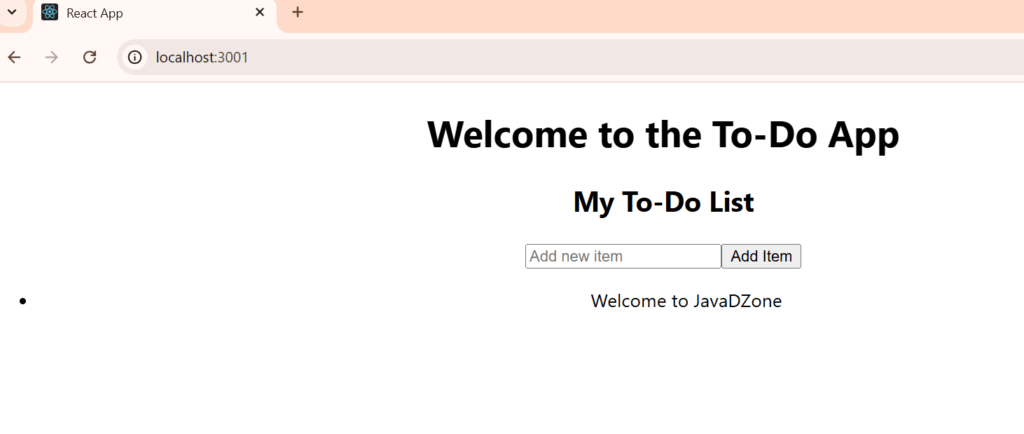
FAQs
1. What is the main purpose of JSX in React?
JSX allows developers to write HTML-like syntax directly in JavaScript, simplifying component structure and improving code readability.
2. Can we use JavaScript functions inside JSX?
Yes! You can use JavaScript functions and expressions within JSX by enclosing them in curly braces {}
.
3. Why do we need to wrap multiple JSX elements in a single parent element?
React components return a single element. Wrapping multiple elements ensures the component structure is cohesive and prevents rendering errors.
4. Is JSX required to write React applications?
While JSX is not mandatory, it’s highly recommended as it simplifies the code and enhances readability.
5. How does React handle JSX under the hood?
JSX is transpiled into React’s React.createElement()
function, which constructs JavaScript objects representing UI elements.
Thank you for reading! If you found this guide helpful and want to stay updated on more React.js content, be sure to follow us for the latest tutorials and insights: JavaDZone React.js Tutorials. Happy coding!