React has revolutionized how we build user interfaces, and understanding its lifecycle methods and hooks is essential for both beginners and experienced developers. In this post, titled React JS Lifecycle Methods and Hooks, we’ll explore the lifecycle of React components, delve into the popular hooks useEffect
and useState
, and provide practical examples to illustrate their use. This guide aims to be both beginner-friendly and insightful for seasoned developers.
What Are Lifecycle Methods?
Lifecycle methods are special functions that allow you to run code at specific points in a component’s life. These methods are particularly useful in class components, enabling you to manage tasks such as data fetching, subscriptions, and cleanup.
Lifecycle Methods in Class Components
In class components, React provides several lifecycle methods:
- Mounting: The component is being created and inserted into the DOM.
componentDidMount()
: Invoked immediately after a component is mounted.
- Updating: The component is being re-rendered due to changes in props or state.
componentDidUpdate(prevProps, prevState)
: Invoked immediately after updating occurs.
- Unmounting: The component is being removed from the DOM.
componentWillUnmount()
: Invoked immediately before a component is unmounted and destroyed.
Example
File Name: LifecycleMethods.js
Create a file named LifecycleMethods.js
and add the following code:
import React from 'react';
class Timer extends React.Component {
constructor(props) {
super(props);
this.state = { seconds: 0 };
}
componentDidMount() {
this.interval = setInterval(() => this.setState({ seconds: this.state.seconds + 1 }), 1000);
}
componentWillUnmount() {
clearInterval(this.interval);
}
render() {
return <div>Seconds: {this.state.seconds}</div>;
}
}
export default Timer;
Output
When you run the above code, you’ll see a timer incrementing every second:
Seconds: 0
Seconds: 1
Seconds: 2
...
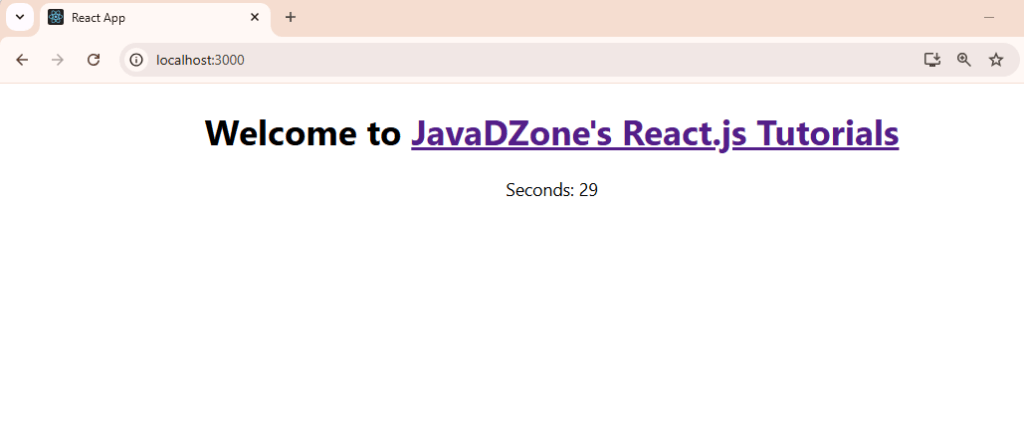
Introduction to React Hooks
React Hooks were introduced in React 16.8 to allow functional components to manage state and side effects without using classes. This makes components simpler and easier to read.
Understanding useState
The useState
hook allows you to add state to functional components.
Example
File Name: Counter.js
Create a file named Counter.js
and add the following code:
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
export default Counter;
Output
When you run the above code, you will see a button that updates the count every time it is clicked:
You clicked 0 times
[Click me button]
Clicking the button will update the count, e.g., You clicked 1 times
, You clicked 2 times
, etc.
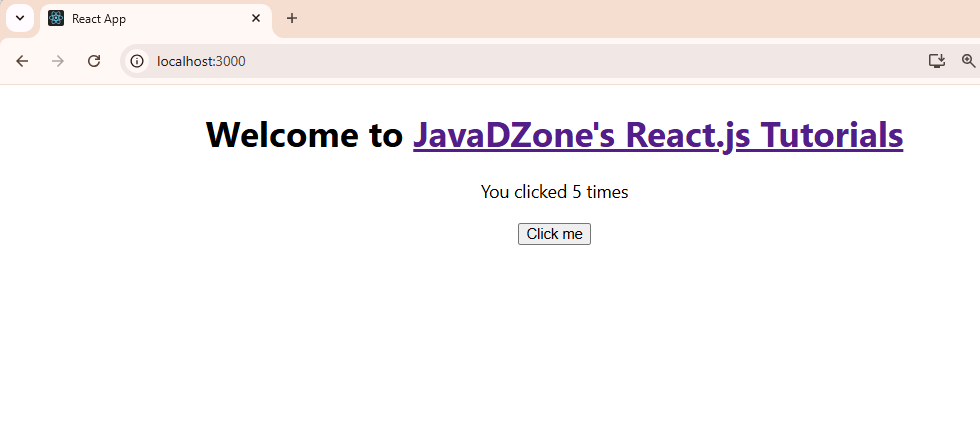
Understanding useEffect
The useEffect
hook manages side effects in functional components, such as data fetching, subscriptions, or manually changing the DOM.
Example
File Name: FetchData.js
Create a file named FetchData.js
and add the following code:
import React, { useState, useEffect } from 'react';
function FetchData() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []); // The empty array ensures this runs only once (like componentDidMount)
return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>;
}
export default FetchData;
Output
When you run this component, you’ll initially see “Loading…”. Once the data is fetched from the API, it will display the fetched data in JSON format.
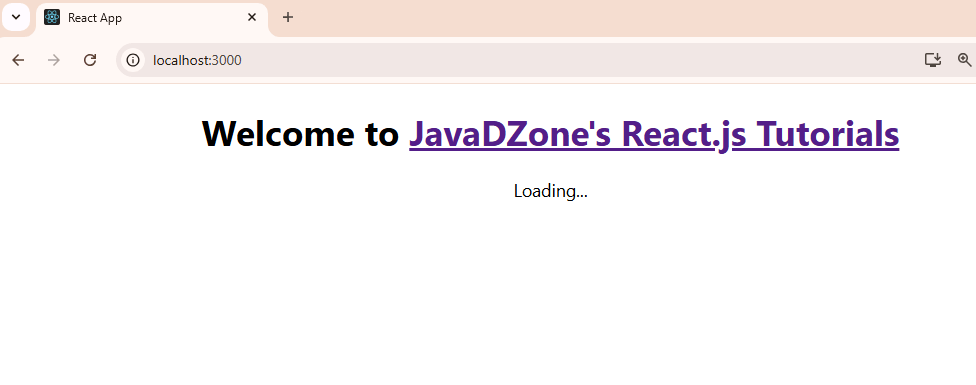
Best Practices for Lifecycle Methods and Hooks
- Keep Side Effects in useEffect: Always use
useEffect
for side effects in functional components to maintain a clean separation of concerns. - Cleanup Functions: If your effect creates a subscription or some resource, ensure you return a cleanup function to prevent memory leaks.
- Dependency Arrays: Always specify dependencies in the
useEffect
hook to avoid unexpected behavior. If your effect relies on specific props or state, list them in the array. - Functional Updates with useState: When updating state based on the previous state, use the functional form to ensure you have the most current state.
Example of Best Practices
File Name: BestPractices.js
Create a file named BestPractices.js
and add the following code:
import React, { useEffect } from 'react';
function WindowSize() {
useEffect(() => {
const handleResize = () => {
console.log(window.innerWidth);
};
window.addEventListener('resize', handleResize);
// Cleanup
return () => window.removeEventListener('resize', handleResize);
}, []);
return <div>Resize the window to see console logs</div>;
}
export default WindowSize;
Output
When you run this component, it will log the window width to the console every time you resize the window.
Resize the window to see console logs
FAQs
1. What is the difference between class components and functional components with hooks?
Class components use lifecycle methods to manage state and side effects, while functional components with hooks use hooks like useState
and useEffect
for the same purposes, leading to cleaner and more concise code.
2. Can I use hooks in class components?
No, hooks are designed for functional components only. If you need state or lifecycle methods in a class component, you must use lifecycle methods.
3. How do I handle multiple state variables?
You can call useState
multiple times to manage different state variables. For example:
const [count, setCount] = useState(0);
const [name, setName] = useState('');
4. What happens if I don’t provide a dependency array in useEffect?
If you don’t provide a dependency array, the effect will run after every render, which can lead to performance issues and infinite loops if not handled properly.
5. Can I use useEffect for data fetching?
Yes, useEffect
is perfect for data fetching and can be used to manage the loading state as well.
Conclusion
Mastering lifecycle methods and React hooks is vital for creating efficient and maintainable React applications. By following best practices and utilizing these features effectively, you can enhance your development workflow and improve your application’s performance.
Thank you for reading! If you found this guide helpful and want to stay updated on more React.js content, be sure to follow us for the latest tutorials and insights: JavaDZone React.js Tutorials. Happy coding!