Handling events in React JS is a fundamental skill for any developer, whether you’re just starting or you’ve been working with React JS for years. Mastering event handling in React JS not only makes your applications more dynamic and interactive but also lays a strong foundation for creating more complex features.
In this article, we’ll cover everything you need to know about event handling in React, including practical examples, best practices, and FAQs. This guide is designed to be beginner-friendly but also provides advanced insights for experienced developers.
Table of Contents
1. Introduction to Event Handling in React JS
In React, event handling is similar to handling events in plain HTML and JavaScript, but with some unique differences. React uses what is called a synthetic event system, which helps maintain cross-browser compatibility. Events are a core part of React applications, enabling interactivity by responding to user actions like clicks, keypresses, form submissions, and more.
2. Understanding Event Basics in React
In HTML, you’d typically write event listeners directly within the element:
<button onclick="handleClick()">Click Me</button>
However, in React, events are handled a bit differently, using camelCase for naming and attaching functions directly:
<button onClick={handleClick}>Click Me</button>
Example: App.js
// Import React
import React from 'react';
// Define the functional component
function App() {
const handleClick = () => {
alert("Button clicked!");
};
return (
<div>
<button onClick={handleClick}>Click Me</button>
</div>
);
}
export default App;
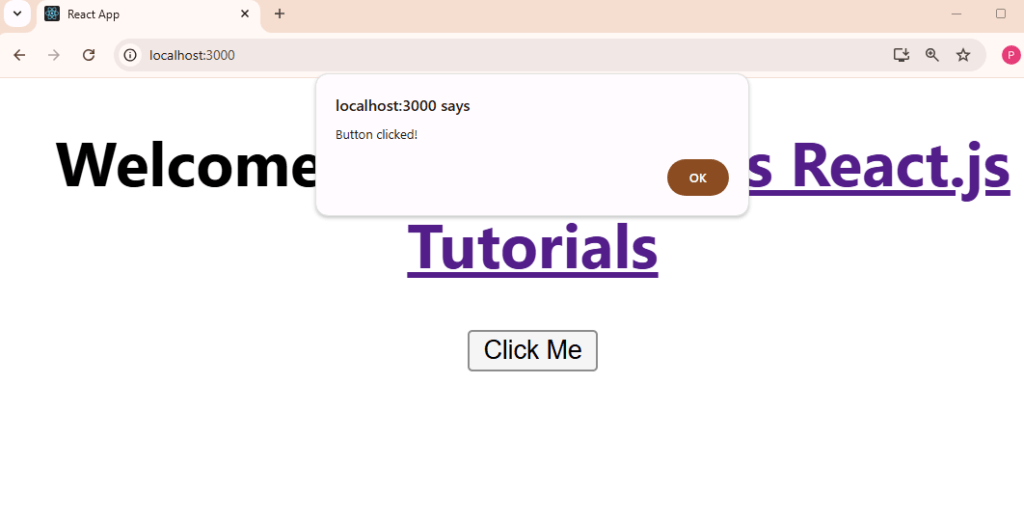
3. Event Binding in React
In React class components, you may encounter event binding issues due to JavaScript’s this
keyword. There are several ways to bind events in React, especially when working with class components.
Binding in the Constructor
Example: App.js
class App extends React.Component {
constructor(props) {
super(props);
this.state = { message: "Hello!" };
this.handleClick = this.handleClick.bind(this);
}
handleClick() {
this.setState({ message: "Button clicked!" });
}
render() {
return (
<div>
<button onClick={this.handleClick}>Click Me</button>
<p>{this.state.message}</p>
</div>
);
}
}
Using Arrow Functions
Arrow functions automatically bind the this
context.
handleClick = () => {
this.setState({ message: "Button clicked!" });
}
4. Passing Arguments to Event Handlers
Sometimes, you may need to pass parameters to event handlers. You can do this by wrapping the handler in an inline arrow function.
Example: App.js
function App() {
const handleClick = (message) => {
alert(message);
};
return (
<div>
<button onClick={() => handleClick("Button clicked!")}>Click Me</button>
</div>
);
}
5. Synthetic Events in React
React provides a cross-browser wrapper called SyntheticEvent for native events. This wrapper offers consistent behavior across different browsers. Synthetic events work the same as native events but come with additional benefits, such as performance optimizations by React.
6. Practical Examples of Common Events
Here are some frequently used events in React and how to implement them:
1. onChange Event
Commonly used with input elements to handle form data.
function App() {
const handleChange = (event) => {
console.log("Input value:", event.target.value);
};
return (
<input type="text" onChange={handleChange} placeholder="Type here..." />
);
}
2. onSubmit Event
Typically used with forms.
function App() {
const handleSubmit = (event) => {
event.preventDefault();
alert("Form submitted!");
};
return (
<form onSubmit={handleSubmit}>
<button type="submit">Submit</button>
</form>
);
}
3. onMouseEnter and onMouseLeave Events
Used to detect when a user hovers over an element.
function App() {
const handleMouseEnter = () => console.log("Mouse entered");
const handleMouseLeave = () => console.log("Mouse left");
return (
<div
onMouseEnter={handleMouseEnter}
onMouseLeave={handleMouseLeave}
style={{ padding: "20px", border: "1px solid #ddd" }}
>
Hover over me
</div>
);
}
7. Best Practices for Event Handling
- Use Arrow Functions Carefully: Avoid using arrow functions directly in JSX to prevent unnecessary re-renders.
- Optimize for Performance: For performance-sensitive code, use
React.memo
to prevent re-renders and event handling issues. - Event Delegation: In lists or dynamic content, consider using event delegation to manage events more efficiently.
- Avoid Inline Functions: Avoid inline functions when possible, as they can lead to unnecessary re-renders and reduced performance.
8. FAQs
Q1: What are synthetic events in React?
A: Synthetic events in React are wrappers around native events, providing consistent behavior across browsers. They ensure better performance and cross-browser compatibility.
Q2: How do I prevent the default behavior of an event in React?
A: Use event.preventDefault()
in the event handler function to prevent the default behavior. For example:
function handleSubmit(event) {
event.preventDefault();
// custom code here
}
Q3: How do I pass arguments to an event handler in React?
A: Wrap the handler in an arrow function and pass the arguments as needed:
<button onClick={() => handleClick("argument")}>Click Me</button>
Thank you for reading! If you found this guide helpful and want to stay updated on more React.js content, be sure to follow us for the latest tutorials and insights: JavaDZone React.js Tutorials. Happy coding!