React.js has revolutionized the way we build user interfaces, and understanding its core concepts is crucial for developers of all levels. One such concept is the difference between controlled and uncontrolled components in react js. In this post, we’ll dive deep into these two types of components, their use cases, and best practices. By the end, you’ll have a solid grasp of how to implement them effectively in your projects.
Controlled and Uncontrolled Components in React JS
What Are Controlled Components
Controlled components are those where the form data is handled by the state of the component. In simpler terms, the React component maintains the current state of the input fields, and any changes to these fields are managed via React’s state management.
How Controlled Components Work
In a controlled component, the input’s value is determined by the state of the component. This means that every time the user types in an input field, the component’s state is updated, and the rendered input value reflects that state.
Example of a Controlled Component
Create ControlledComponent.js
import React, { useState } from 'react';
function ControlledComponent() {
const [inputValue, setInputValue] = useState('');
const handleChange = (event) => {
setInputValue(event.target.value);
};
return (
<div>
<input
type="text"
value={inputValue}
onChange={handleChange}
/>
<p>You typed: {inputValue}</p>
</div>
);
}
export default ControlledComponent;
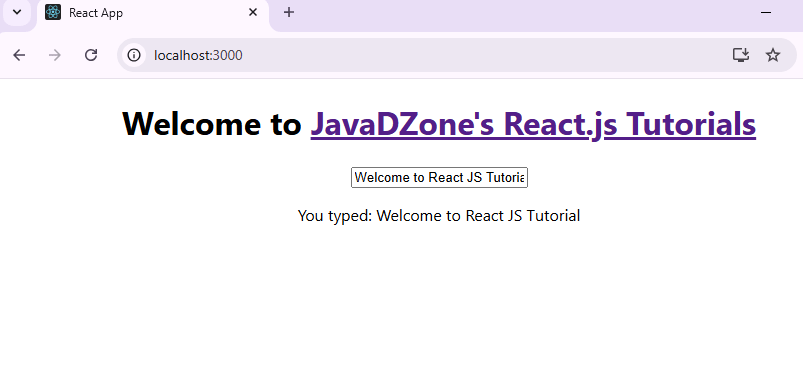
Best Practices for Controlled Components
- Always use state: Keep your input values in the component state to make them easily accessible and modifiable.
- Validate Input: Implement validation logic in the
handleChange
function to ensure that the input meets your requirements. - Form Submission: When using controlled components in forms, prevent the default form submission to handle the input data in a React-friendly way.
What Are Uncontrolled Components?
Uncontrolled components, on the other hand, are components that store their form data in the DOM instead of the component’s state. This means that when you want to access the input values, you use refs to get the current values directly from the DOM.
How Uncontrolled Components Work
With uncontrolled components, you don’t need to update the state on every input change. Instead, you can access the current value when needed, such as during form submission.
Example of an Uncontrolled Component
Create UncontrolledComponent.js
import React, { useRef } from 'react';
function UncontrolledComponent() {
const inputRef = useRef(null);
const handleSubmit = (event) => {
event.preventDefault();
alert('You typed: ' + inputRef.current.value);
};
return (
<form onSubmit={handleSubmit}>
<input type="text" ref={inputRef} />
<button type="submit">Submit</button>
</form>
);
}
export default UncontrolledComponent;
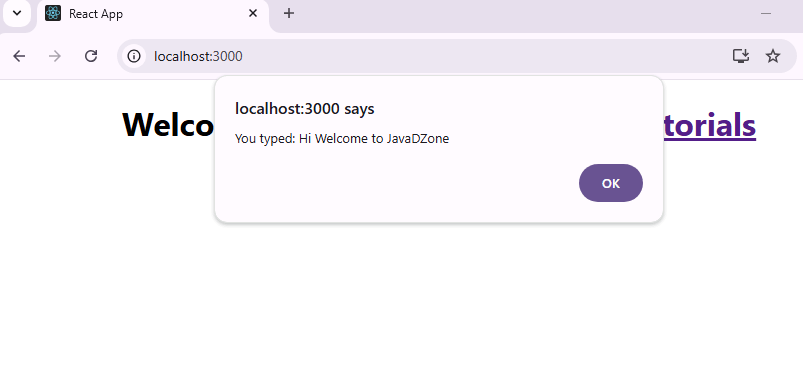
Best Practices for Uncontrolled Components
- Use refs sparingly: Uncontrolled components can lead to less predictable behavior, so use them only when necessary.
- Access DOM elements directly: Use refs to get the current values at specific moments (like form submission), rather than keeping them in state.
- Combine with controlled components when needed: For complex forms, a mix of both approaches can be beneficial.
When to Use Controlled vs. Uncontrolled Components
- Controlled Components: Ideal for scenarios where you need to validate inputs, manage form state, or react to user input dynamically.
- Uncontrolled Components: Suitable for simple forms where performance is a concern, or you want to integrate with non-React codebases.
Conclusion
Understanding controlled and uncontrolled components is fundamental for any React developer. Controlled components provide more control over the input data, making them a great choice for complex forms, while uncontrolled components offer a simpler and more performant option for straightforward use cases.
FAQ
1. Can I convert a controlled component to an uncontrolled one?
Yes, you can convert by removing state management and using refs for input value retrieval.
2. Are uncontrolled components less efficient?
Not necessarily, but they can make your component’s behavior less predictable since they rely on the DOM.
3. Can I mix controlled and uncontrolled components?
Yes, it’s common to use both within a single form, depending on the requirements.
4. What are some libraries that work well with controlled components?
Libraries like Formik and React Hook Form are designed to work with controlled components and can simplify form management.
Thank you for reading! If you found this guide helpful and want to stay updated on more React.js content, be sure to follow us for the latest tutorials and insights: JavaDZone React.js Tutorials. Happy coding!