Introduction
React is a powerful JavaScript library for building user interfaces, especially single-page applications where data changes over time. As your application grows, managing state effectively becomes crucial. Two of the most essential tools for handling complex state in React Reducers and Context API.
In this blog, we will dive into what Reducers and Context API are, how they work together, and when to use Reducers effectively. This guide is designed to help both beginners and experienced developers with practical examples and best practices.
Table of Contents
- What is Context API?
- What is a Reducer?
- Context API vs. Props
- When to Use Reducers in React
- Setting Up a Project
- Example: Using Context API with Reducers
- Best Practices for Using Reducers
- FAQs
React Reducers and Context API
1. What is Context API?
The Context API is a React feature introduced in version 16.3, designed to help with prop drilling issues. When you need to pass data through multiple nested components, the Context API allows you to share data without explicitly passing props through each component level.
Use Case: For themes, user authentication, and language preferences, Context API can manage global state effectively.
Creating a Context:
File: src/context/ThemeContext.js
2. What is a Reducer?
A Reducer is a function that determines changes to an application’s state. It uses a concept from functional programming and is central to Redux, but can also be used directly in React applications via the useReducer
hook.
Syntax of a Reducer:
A reducer takes the current state and an action as arguments, then returns a new state.
3. Context API vs. Props
- Props are great for passing data from parent to child components. However, when data needs to be accessed by many nested components, prop drilling becomes an issue.
- Context API eliminates the need for prop drilling by providing a way to share data globally across the component tree.
4. When to Use Reducers in React
Use reducers when:
- Complex State Logic: If the state logic is complex and involves multiple sub-values or deep updates, reducers are a good choice.
- State Based on Previous State: When the next state depends on the previous state, reducers help manage state transitions clearly.
- Centralized State Management: For managing centralized state across multiple components, reducers work well in combination with the Context API.
5. Setting Up a Project
Project Structure:
6. Example: Using Context API with Reducers
Step 1: Define the Reducer
File: src/reducers/counterReducer.js
Step 2: Create Context with Reducer
File: src/context/CounterContext.js
Step 3: Create a Counter Component
File: src/components/Counter.js
Step 4: Integrate in App Component
File: src/App.js
Start the application by running the command npm start
.
Output:
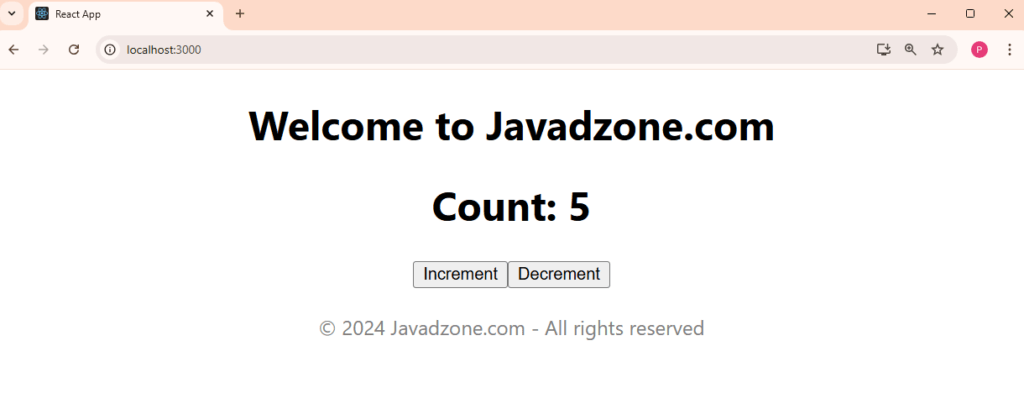
7. Best Practices for Using Reducers
- Keep Reducer Functions Pure: Reducers should be pure functions with no side effects. This makes them predictable and testable.
- Define Action Types: Use constants for action types to avoid typos and make the code more maintainable.
- Organize Files: Separate reducers and context into dedicated folders to keep your project structure clean.
- Use
useReducer
for Complex State: PreferuseReducer
overuseState
when state logic becomes complex.
8. FAQs
Q1. When should I use useReducer
over useState
?
A1: Use useReducer
when state logic is complex or when the next state depends on the previous state. For simple state management, useState
is sufficient.
Q2. Can I use multiple contexts in a single application?
A2: Yes, you can create multiple contexts to manage different pieces of global state, but be cautious about performance and complexity.
Q3. What are the performance implications of using Context API?
A3: Using Context API with large state objects can cause unnecessary re-renders. Optimize performance by splitting contexts or using React.memo
and useCallback
.
Q4. Is Context API a replacement for Redux?
A4: Not necessarily. Context API is great for small to medium applications. For large apps with more complex state logic, Redux may still be a better option.
Q5. Can I use useReducer
without Context API?
A5: Yes, useReducer
can be used independently in a single component for managing complex state logic.
Thank you for reading! If you found this guide helpful and want to stay updated on more React.js content, be sure to follow us for the latest tutorials and insights: JavaDZone React.js Tutorials. Happy coding!