Introduction
Java is a powerful language with numerous features that can enhance your coding experience. This post, titled “Discovering Java’s Hidden Features for Better Code,” uncovers lesser-known Java features to help you write better and more efficient code.
Table of Contents
1. Optional.ofNullable for Safer Null Handling
Avoid NullPointerExceptions using Optional.ofNullable
.
Example:
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
String value = null;
Optional<String> optionalValue = Optional.ofNullable(value);
optionalValue.ifPresentOrElse(
v -> System.out.println("Value is: " + v),
() -> System.out.println("Value is absent")
);
}
}
Output:
Value is absent
In this example, Optional.ofNullable
checks if value
is null and allows us to handle it without explicit null checks.
2. Using Streams for Simplified Data Manipulation
Java Streams API offers a concise way to perform operations on collections.
Advanced Stream Operations:
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Edward");
// Filter and Collect
List<String> filteredNames = names.stream()
.filter(name -> name.length() > 3)
.collect(Collectors.toList());
System.out.println("Filtered Names: " + filteredNames);
// Grouping by length
Map<Integer, List<String>> groupedByLength = names.stream()
.collect(Collectors.groupingBy(String::length));
System.out.println("Grouped by Length: " + groupedByLength);
}
}
Output:
Filtered Names: [Alice, Charlie, David, Edward]
Grouped by Length: {3=[Bob], 5=[Alice, David], 7=[Charlie, Edward]}
This demonstrates filtering a list and grouping by string length using streams, simplifying complex data manipulations.
3. Pattern Matching for Instanceof: Simplifying Type Checks
Introduced in Java 16, pattern matching for instanceof
simplifies type checks and casts.
Real-World Example:
public class InstanceofExample {
public static void main(String[] args) {
Object obj = "Hello, World!";
if (obj instanceof String s) {
System.out.println("The string length is: " + s.length());
} else {
System.out.println("Not a string");
}
}
}
Output:
The string length is: 13
Pattern matching reduces boilerplate code and enhances readability by combining type check and cast in one step.
4. Compact Number Formatting for Readable Outputs
Java 12 introduced compact number formatting, ideal for displaying numbers in a human-readable format.
Example Usage:
import java.text.NumberFormat;
import java.util.Locale;
public class CompactNumberFormatExample {
public static void main(String[] args) {
NumberFormat compactFormatter = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.SHORT);
String result = compactFormatter.format(1234567);
System.out.println("Compact format: " + result);
}
}
Output:
Compact format: 1.2M
This feature is useful for presenting large numbers in a concise and understandable manner, suitable for dashboards and reports.
5. Text Blocks for Clearer Multi-line Strings
Java 13 introduced text blocks, simplifying the handling of multi-line strings like HTML, SQL, and JSON.
Example Usage:
public class TextBlockExample {
public static void main(String[] args) {
String html = """
<html>
<body>
<h1>Hello, World!</h1>
</body>
</html>
""";
System.out.println(html);
}
}
Output:
<html>
<body>
<h1>Hello, World!</h1>
</body>
</html>
Text blocks improve code readability by preserving the formatting of multi-line strings, making them easier to maintain and understand.
6. Unlocking Java’s Concurrent Utilities for Efficient Multithreading
The java.util.concurrent
package offers robust utilities for concurrent programming, enhancing efficiency and thread safety.
Example Usage:
import java.util.Queue;
import java.util.concurrent.ConcurrentLinkedQueue;
public class ConcurrentLinkedQueueExample {
public static void main(String[] args) {
Queue<String> queue = new ConcurrentLinkedQueue<>();
// Adding elements
queue.add("Element1");
queue.add("Element2");
// Polling elements
System.out.println("Polled: " + queue.poll());
System.out.println("Polled: " + queue.poll());
}
}
Output:
Polled: Element1
Polled: Element2
ConcurrentLinkedQueue
is a thread-safe collection, ideal for concurrent applications where multiple threads access a shared collection.
7. Performance Tuning with Java Flight Recorder (JFR)
Java Flight Recorder (JFR) is a built-in feature of Oracle JDK and OpenJDK that provides profiling and diagnostic tools for optimizing Java applications.
Example Usage:
public class JFRDemo {
public static void main(String[] args) throws InterruptedException {
// Enable Java Flight Recorder (JFR)
enableJFR();
// Simulate application workload
for (int i = 0; i < 1000000; i++) {
String result = processRequest("Request " + i);
System.out.println("Processed: " + result);
}
// Disable Java Flight Recorder (JFR)
disableJFR();
}
private static String processRequest(String request) {
// Simulate processing time
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Processed " + request;
}
private static void enableJFR() {
// Code to enable JFR
// Example: -XX:+UnlockCommercialFeatures -XX:+FlightRecorder
}
private static void disableJFR() {
// Code to disable JFR
// Example: -XX:-FlightRecorder
}
}
Explanation:
- Enabling JFR: Configure JVM arguments like
-XX:+UnlockCommercialFeatures -XX:+FlightRecorder
to enable JFR. This allows JFR to monitor application performance metrics. - Simulating Workload: The
processRequest
method simulates a workload, such as handling requests. JFR captures data on CPU usage, memory allocation, and method profiling during this simulation. - Disabling JFR: After monitoring, disable JFR using
-XX:-FlightRecorder
to avoid overhead in production environments.
Java Flight Recorder captures detailed runtime information, including method profiling and garbage collection statistics, aiding in performance tuning and troubleshooting.
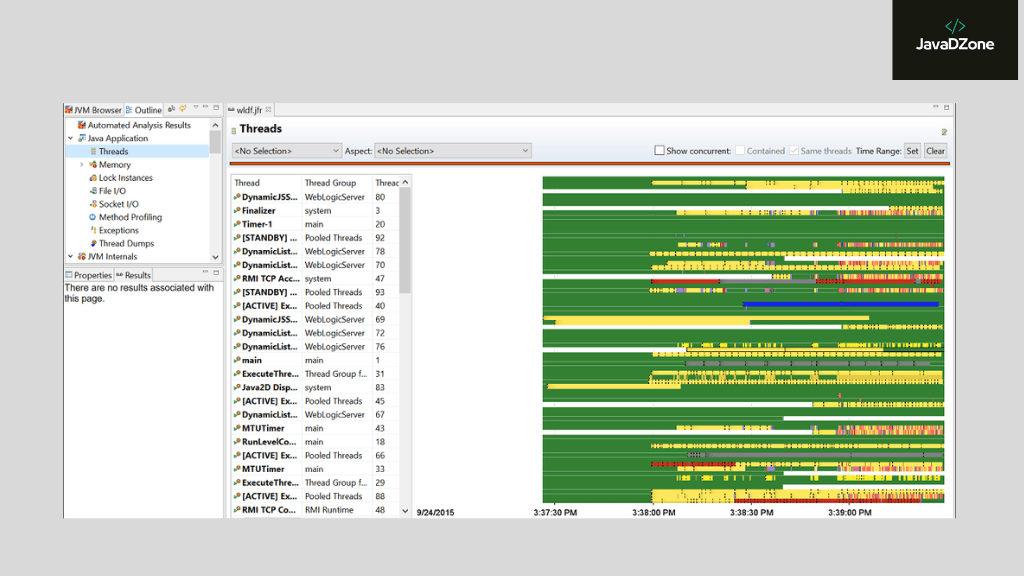
8. Leveraging Method Handles for Efficient Reflection-Like Operations
Method handles provide a flexible and performant alternative to Java’s reflection API for method invocation and field access.
Before: How We Used to Code with Reflection
Before method handles were introduced, Java developers typically used reflection for dynamic method invocation. Here’s a simplified example of using reflection:
import java.lang.reflect.Method;
public class ReflectionExample {
public static void main(String[] args) throws Exception {
String str = "Hello, World!";
Method method = String.class.getMethod("substring", int.class, int.class);
String result = (String) method.invoke(str, 7, 12);
System.out.println(result); // Output: World
}
}
Reflection involves obtaining Method
objects, which can be slower due to runtime introspection and type checks.
With Method Handles: Enhanced Performance and Flexibility
Method handles offer a more direct and efficient way to perform dynamic method invocations:
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodType;
public class MethodHandlesExample {
public static void main(String[] args) throws Throwable {
MethodHandles.Lookup lookup = MethodHandles.lookup();
MethodHandle mh = lookup.findVirtual(String.class, "substring", MethodType.methodType(String.class, int.class, int.class));
String result = (String) mh.invokeExact("Hello, World!", 7, 12);
System.out.println(result); // Output: World
}
}
Output:
World
Method handles enable direct access to methods and fields, offering better performance compared to traditional reflection.
9. Discovering Java’s Hidden Features for Better Code:
Enhanced Date and Time Handling with java.time
Java 8 introduced the java.time
package, providing a modern API for date and time manipulation, addressing shortcomings of java.util.Date
and java.util.Calendar
.
Example Usage:
import java.time.LocalDate;
import java.time.LocalTime;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeExample {
public static void main(String[] args) {
LocalDate date = LocalDate.now();
LocalTime time = LocalTime.now();
LocalDateTime dateTime = LocalDateTime.now();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = dateTime.format(formatter);
System.out.println("Current Date: " + date);
System.out.println("Current Time: " + time);
System.out.println("Formatted Date-Time: " + formattedDateTime);
}
}
Output:
Current Date: 2024-06-15
Current Time: 14:23:45.123
Formatted Date-Time: 2024-06-15 14:23:45
The java.time
API simplifies date and time handling with immutable and thread-safe classes, supporting various date-time operations and formatting.
Conclusion
By leveraging these hidden gems in Java, you can streamline your code, enhance performance, and simplify complex tasks. These features not only improve productivity but also contribute to writing cleaner, more maintainable Java applications. Embrace these tools and techniques to stay ahead in your Java development journey!