Today, we’ll explore how to create a custom starter with Spring Boot 3. This custom starter simplifies the setup and configuration process across different projects. By developing a custom starter, you can package common configurations and dependencies, ensuring they’re easily reusable in various Spring Boot applications. We’ll guide you through each step of creating and integrating this starter, harnessing the robust auto-configuration and dependency management features of Spring Boot.
Benefits of Creating Custom Starters:
- Modularity and Reusability:
- Custom starters encapsulate reusable configuration, dependencies, and setup logic into a single module. This promotes modularity by isolating specific functionalities, making it easier to reuse across different projects.
- Consistency and Standardization:
- By defining a custom starter, developers can enforce standardized practices and configurations across their applications. This ensures consistency in how components like databases, messaging systems, or integrations are configured and used.
- Reduced Boilerplate Code:
- Custom starters eliminate repetitive setup tasks and boilerplate code. Developers can quickly bootstrap new projects by simply including the starter dependency, rather than manually configuring each component from scratch.
- Simplified Maintenance:
- Centralizing configuration and dependencies in a custom starter simplifies maintenance. Updates or changes to common functionalities can be made in one place, benefiting all projects that use the starter.
- Developer Productivity:
- Developers spend less time on initial setup and configuration, focusing more on implementing business logic and features. This accelerates development cycles and enhances productivity.
Step 1: Setting Up the Custom Messaging Starter
Let’s start by setting up a new Maven project named custom-messaging-starter
.
- Setting Up the Maven ProjectCreate a new directory structure for your Maven project:
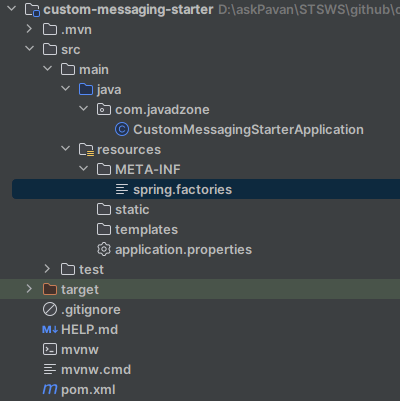
2. Define Dependencies and Configuration
Update the pom.xml
file with necessary dependencies like spring-boot-starter
and spring-boot-starter-amqp
. This helps in managing RabbitMQ connections seamlessly.
3. Creating Auto-Configuration
Develop the CustomMessagingAutoConfiguration
class to configure RabbitMQ connections. This ensures that messaging between microservices is streamlined without manual setup.
4. Registering Auto-Configuration
Create a META-INF/spring.factories
file within src/main/resources
to register your auto-configuration class:
5. Building and Installing
Build and install your custom starter into the local Maven repository using the following command:
Step 2: Using the Custom Messaging Starter in a Spring Boot Application
Now, let’s see how you can utilize this custom messaging starter (custom-messaging-starter
) in a Spring Boot application (my-messaging-app
).
- Adding DependencyInclude the custom messaging starter dependency in the
pom.xml
file of your Spring Boot application:
2. Configuring RabbitMQ
Configure RabbitMQ connection properties in src/main/resources/application.properties
:
3. Using RabbitTemplate
Implement a simple application to send and receive messages using RabbitMQ:
4. Setting Up a Listener
To demonstrate message receiving, add a listener component:
When to Create Custom Starter with Spring Boot 3 in Real-Time Applications:
- Complex Configuration Requirements:
- When an application requires complex or specialized configurations that are consistent across multiple projects (e.g., database settings, messaging queues), a custom starter can abstract these configurations for easy integration.
- Cross-Project Consistency:
- Organizations with multiple projects or microservices can use custom starters to enforce consistent practices and configurations, ensuring uniformity in how applications are developed and maintained.
- Encapsulation of Best Practices:
- If your organization has established best practices or patterns for specific functionalities (e.g., logging, security, caching), encapsulating these practices in a custom starter ensures they are applied uniformly across applications.
- Third-Party Integrations:
- Custom starters are beneficial when integrating with third-party services or APIs. They can encapsulate authentication methods, error handling strategies, and other integration specifics, simplifying the integration process for developers.
- Team Collaboration and Knowledge Sharing:
- Creating custom starters promotes collaboration among teams by standardizing development practices. It also serves as a knowledge-sharing tool, allowing teams to document and share common configurations and setups.
Conclusion
By creating a custom Spring Boot starter for messaging with RabbitMQ, you streamline configuration management across projects. This encapsulation ensures consistency in messaging setups, reduces redundancy, and simplifies maintenance efforts. Custom starters are powerful tools for enhancing developer productivity and ensuring standardized practices in enterprise applications.
Related Articles:
- What is Spring Boot and Its Features
- Spring Boot Starter
- Spring Boot Packaging
- Spring Boot Custom Banner
- 5 Ways to Run Spring Boot Application
- @ConfigurationProperties Example: 5 Proven Steps to Optimize
- Mastering Spring Boot Events: 5 Best Practices
- Spring Boot Profiles Mastery: 5 Proven Tips
- CommandLineRunners vs ApplicationRunners
- Spring Boot Actuator: 5 Performance Boost Tips
- Spring Boot API Gateway Tutorial
- Apache Kafka Tutorial
- Spring Boot MongoDB CRUD Application Example
- ChatGPT Integration with Spring Boot
- RestClient in Spring 6.1 with Examples
- Spring Boot Annotations Best Practices