The Spring Cloud Config Server: Decentralized Configuration Management
The Spring Cloud Config Server empowers us to extract our microservice application’s configuration to an external repository and distribute it across the network in a decentralized manner. This decentralization facilitates convenient accessibility.
Table of Contents
Advantages of spring cloud config server
Utilizing the Spring Cloud Config Server offers a significant advantage: the ability to modify service configurations externally, without necessitating changes to the application source code. This circumvents the need to rebuild, repackage, and redeploy the microservice application across various cluster nodes.
Embedding application configuration within the application itself can lead to several complications:
- Rebuilding for Configuration Changes: Each configuration change requires rebuilding the application, yielding a new artifact version (jar).
- Containerized Environments: In containerized environments, producing and publishing new versions of containerized images (e.g., Docker images) becomes necessary.
- Complex Redeployment Process: Identifying running service instances, stopping, redeploying the new service version, and restarting it becomes a complex and time-consuming endeavor, involving multiple teams.
- Real-Time Configuration Updates: The Spring Cloud Config Server enables configurations to be updated in real time without service interruption, enhancing agility in response to changing requirements.
- Centralized Management: All configurations can be centrally managed and versioned, ensuring consistency and streamlined change tracking.
- Decoupling Configurations: By externalizing configurations, services are detached from their configuration sources, simplifying the independent management of configurations.
- Consistency Across Environments: The Config Server guarantees uniform configurations across various environments (development, testing, production), reducing discrepancies and errors.
- Rollback and Auditing: With version control and historical tracking, reverting configurations and auditing changes becomes seamless.
- Enhanced Security and Access Control: The Config Server incorporates security features for controlling access to and modification of configurations, reinforcing data protection.
Spring Cloud: A Solution for Easier Configuration Management
To address these challenges, Spring introduces the Spring Cloud module, encompassing the Config Server and Config Client tools. These tools aid in externalizing application configuration in a distributed manner, streamlining configuration management. This approach delivers the following benefits:
- The ConfigServer/ConfigClient tools facilitate the externalization of application configuration in a distributed fashion.
- Configuration can reside in a Git repository location, obviating the need to embed it within the application.
- This approach expedites configuration management and simplifies the process of deploying and maintaining the application.
By adopting the ConfigServer and ConfigClient tools, Spring Cloud simplifies the management of application configuration, enhancing efficiency and minimizing the time and effort required for deployment and maintenance.
Building Spring Cloud Config Server Example
To build the Spring Cloud Config Server, you can use Maven/gradle as your build tool. Below is the pom.xml
file containing the necessary dependencies and configuration for building the config server:
Spring Cloud Config Server Dependency
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-config-server</artifactId>
</dependency>
</dependency>
Activating Spring Cloud Config Server Within Your Spring Boot App
@EnableConfigServer
@SpringBootApplication
public class CloudConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(CloudConfigServerApplication.class, args);
}
}
By adding the @EnableConfigServer
annotation, you activate the Spring Cloud Config Server features within your application.
Include the following configurations in the properties
To configure your Spring Cloud Config Server, you can make use of the application.properties
file. For instance:
server.port=8888
spring.application.name=cloud-config-server
server.servlet.context-path=/api/v1
Customizing Configuration Search Locations with application-native.properties
Furthermore, you can include configurations specific to the application-native.properties
file. If your configuration client searches for configurations in the classpath’s /configs
folder, you can specify this in the properties:
- Create an
application-native.properties
file in theresources
folder. - Include the following configuration in the file to define the search locations:
spring.cloud.config.server.native.searchLocations=classpath:/configs,classpath:/configs/{application}
With these configurations in place, your Spring Cloud Config Server will be primed to handle configuration management effectively.
Generate a configuration file using the exact name as the config client application, and craft properties files corresponding to different environments. For instance:
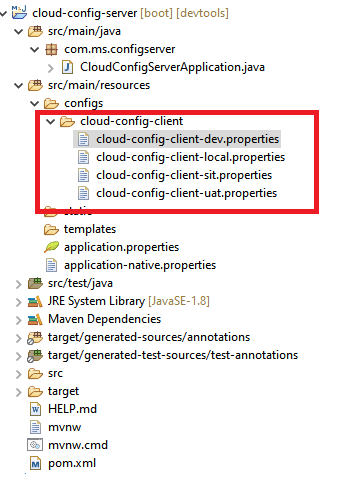
Include the following properties within the cloud-config-client-dev.properties
file. You can adjust the properties according to the specific profiles:
spring.application.name=cloud-config-client
server.port=8080
student.name=Sachin
student.rollNo=1234
student.email=sachin@gmail.com
student.phone=123456789
To initiate the application, provide the subsequent VM argument:
-Dspring.profiles.active=local,native
For further reference, you can access the source code on GitHub at: https://github.com/askPavan/cloud-config-server