React.js, commonly referred to as React, is an open-source JavaScript library created by Facebook in 2013. What is React JS? A Beginner’s Guide to React.js Framework Designed for building interactive, dynamic user interfaces (UI), React has since become one of the most popular choices for web development. React enables developers to build scalable, fast, and efficient applications by breaking down complex UIs into reusable components, which can dramatically simplify both the development and maintenance of applications.
Table of Contents
Why Choose React?
React is favored by many developers due to its flexibility, speed, and efficiency. Here are a few reasons why React is widely adopted:
- Reusable Components: React allows developers to create independent, reusable components that can be used throughout the application. This leads to faster development and consistent user experiences.
- Virtual DOM: Unlike traditional DOM manipulation, React uses a virtual DOM that improves performance by minimizing real DOM changes. This optimizes rendering and enhances the speed of the application.
- Declarative Syntax: React’s declarative syntax makes code more readable and easier to debug. Developers can describe what the UI should look like, and React efficiently manages the underlying updates.
- Large Community and Ecosystem: React has a vibrant community, a vast library of third-party tools, and extensive documentation, making it beginner-friendly while also catering to complex projects.
Setting Up a React Environment
To get started with React, you need Node.js and npm (Node Package Manager) installed on your machine.
- Install Node.js:
- Download and install Node.js from the official website. This will automatically install npm as well.
- Create a New React Application:
- Open your terminal and run the following command:
- Replace
my-app
with your project name. This command sets up a new React project with all the necessary files and dependencies.
3. Start the Development Server:
Navigate into the project folder:
Run the following command to start the application:
Your React app will be running locally at http://localhost:3000.
Understanding React Components and What is React JS
React applications are built with components. Components are small, reusable pieces of code that describe part of the UI.
Example: A Simple Functional Component
This Greeting
component returns a simple heading. To use it in your main app, you can import and render it as follows:
Functional vs Class Components
- Functional Components: These are simpler and are written as JavaScript functions. They became widely used after React introduced Hooks, allowing for state and lifecycle features.
- Class Components: Written as ES6 classes, they were originally the only way to handle component state and lifecycle methods before Hooks.
State and Props in React
- State: A component’s state is an object that holds dynamic data. When state changes, React re-renders the component to reflect the new state.
2. Props: Props (short for “properties”) allow data to be passed from a parent component to a child component.
Best Practices in React
- Keep Components Small and Focused: Each component should have a single purpose to make code more readable and reusable.
- Use Descriptive Names: Name components based on what they represent.
- Avoid Direct DOM Manipulation: Use state and props for any updates instead of directly manipulating the DOM.
- Utilize Hooks: Make use of React’s built-in Hooks, like
useState
,useEffect
, anduseContext
, to manage state and lifecycle events in functional components. - Use Key Props in Lists: If rendering lists, always include a unique
key
prop for each element to enhance performance.
Practical Example: Building a Simple Todo List in React
In this example, we’ll build a simple Todo List application to demonstrate how to use state and events in React. Follow these steps to set up and understand the project structure. This guide will help you know exactly which files to create and where to paste the code.
Step 1: Set Up Your React Project
- Create a New React App:
Open your terminal and run:
Replace my-todo-app
with any name for your project. This command will create a new React project folder with the necessary files.
2. Open the Project: Navigate to the project folder:
Start the development server by running:
This will open the React app at http://localhost:3000
Step 2: Create a New Component for Todo List
- Inside the
src
folder, create a new file calledTodo.js
. This component will handle the core functionality of our Todo List. - Add the Following Code in
Todo.js
: This code creates a simple form where users can add new todo items and displays them in a list.
- Explanation:
- State Management: We use
useState
to store the list of todos and the current input for a new todo. - Event Handling: The
addTodo
function adds a new todo to the list when the “Add” button is clicked, and it also clears the input field.
- State Management: We use
Step 3: Use the Todo Component in App.js
- Open
App.js
in thesrc
folder. By default,App.js
is the main file that renders components on the page. - Import and Use the
Todo
Component: Add the following code inApp.js
to include the Todo component we created.
3. Save and View:
Save the file, and if your development server is still running, your Todo List app should now appear at http://localhost:3000.
You should see a heading “My React Todo App,” an input field, an “Add” button, and an area where todos will be listed.
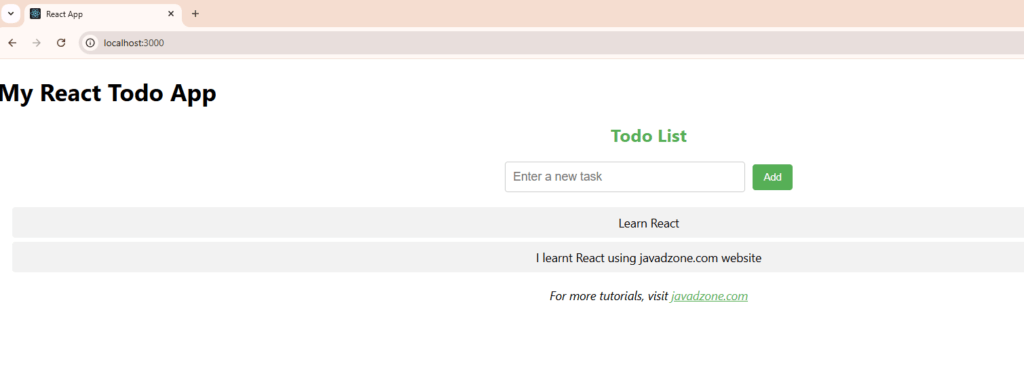
FAQs
1. What is React used for?
React is used to build interactive, dynamic, and responsive web applications by simplifying UI development with reusable components.
2. Do I need to know JavaScript before learning React?
Yes, a basic understanding of JavaScript is essential, as React relies heavily on JavaScript concepts.
3. What are React Hooks?
Hooks, introduced in React 16.8, are functions like useState
and useEffect
that let you use state and other React features in functional components.
4. How is React different from Angular?
While both are used for front-end development, React is a library focused solely on the UI, while Angular is a full-fledged framework offering more structure and tools for building applications.
5. Can I use React with backend frameworks like Node.js?
Yes, React works well with backend frameworks like Node.js to handle the server-side logic and API endpoints.
Thank you for reading! If you found this guide helpful and want to stay updated on more React.js content, be sure to follow us for the latest tutorials and insights: JavaDZone React.js Tutorials. Happy coding!