Java is evolving to make it easier for beginners to start coding without the complexity of large-scale programming. With the introduction of Unnamed Classes in Java 21, this enhancement allows students to write simple programs initially and gradually incorporate more advanced features as they gain experience. This feature aims to simplify the learning curve for newcomers.
Table of Contents
Simplifying a Basic Java Program
Think about a straightforward Java program, like one that calculates the sum of two numbers:
public class AddNumbers {
public static void main(String[] args) {
int num1 = 5;
int num2 = 7;
int sum = num1 + num2;
System.out.println("The sum is: " + sum); //12
}
}
This program may appear more complicated than it needs to be for such a simple task. Here’s why:
- The class declaration and the mandatory public access modifier are typically used for larger programs but are unnecessary here.
- The String[] args parameter is designed for interacting with external components, like the operating system’s shell. However, in this basic program, it serves no purpose and can confuse beginners.
- The use of the static modifier is part of Java’s advanced class-and-object model. For beginners, it can be perplexing. To add more functionality to this program, students must either declare everything as static (which is unconventional) or Learn about static and instance members and how objects are created.
In Java 21, making it easier for beginners to write their very first programs without the need to understand complex features designed for larger applications. This enhancement involves two key changes
1. Instance Main Methods:
The way Java programs are launched is changing, allowing the use of instance main methods. These methods don’t need to be static, public, or have a String[] parameter. This modification enables simplifying the traditional “Hello, World!” program to something like this:
class GreetingProgram {
void greet() {
System.out.println("Hello, World!");
}
}
Execute the program using the following command: java --source 21 --enable-preview GreetingProgram.java
Output
Hello, World!
2. Unnamed Classes:
Java programmers introducing unnamed classes to eliminate the need for explicit class declarations, making the code cleaner and more straightforward:
void main() {
System.out.println("Welcome to Java 21 Features");
}
Save this file with a name of your choice, then run the program using the following command: java --source 21 --enable-preview YourFileName.java
Ensure that you replace “YourFileName” with the actual name of your file.
Output:
Welcome to Java 21 Features
Please find the reference output below.
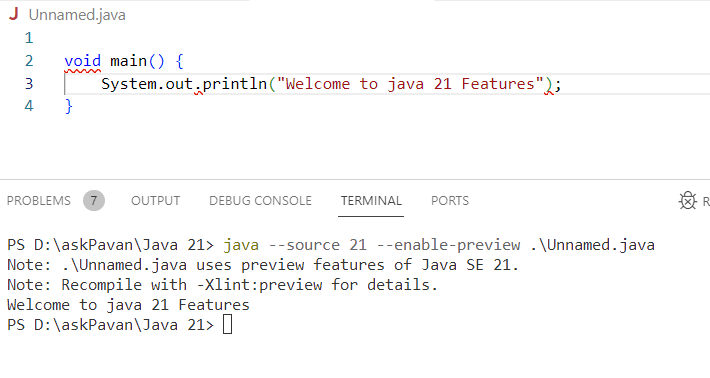
Please note that these changes are part of a preview language feature, and they are disabled by default. To try them out in JDK 21, you can enable preview features using the following commands:
Compile the program with: javac --release 21 --enable-preview FileName.java
run it with: java --source 21 --enable-preview FileName.java
You can find more information about these features on the official OpenJDK website by visiting the following link: Java Enhancement Proposal 443 (JEP 443).
To sum it up, Java 21 is bringing some fantastic improvements to make programming more beginner-friendly and code cleaner. With the introduction of instance main methods and unnamed classes, Java becomes more accessible while maintaining its strength. These changes mark an exciting milestone in Java’s evolution, making it easier for newcomers to dive into coding. Since these features are in preview, developers have the opportunity to explore and influence the future of Java programming.