How to set up a React development environment correctly is essential for a smooth and productive development experience. In this guide, we’ll explore the steps for setting up Node.js, NPM, and creating a new React app. We’ll cover the best practices, explain why each step matters, and share practical examples to help both beginners and experienced developers.
1. What is React?
React is a popular JavaScript library for building user interfaces, created and maintained by Facebook. Its component-based structure allows developers to build efficient, reusable, and maintainable UIs. React is commonly used for developing single-page applications (SPAs) where the user experience is fluid, responsive, and interactive.
2. Why Node.js and NPM?
Node.js and NPM are essential tools for working with React:
- Node.js is a JavaScript runtime that allows you to run JavaScript on your server or local machine, enabling the use of tools like React.
- NPM (Node Package Manager) helps you manage JavaScript packages and dependencies, making it easy to install libraries and keep your project up-to-date.
By using Node.js and NPM, you can streamline the setup and maintenance of a React environment.
3. System Requirements
To start, make sure your computer meets the following requirements:
- Operating System: Windows, macOS, or Linux
- RAM: 4GB or more recommended
- Disk Space: 500MB free for Node.js and NPM installation
- Text Editor: Visual Studio Code, Atom, or any other preferred editor
How to Set Up a React Development Environment
4. Step-by-Step Setup of Node.js and NPM
Step 1: Download Node.js
- Visit Node.js’s official website.
- Download the LTS (Long Term Support) version for stability and compatibility.
- Run the installer and follow the instructions. Check the box to install NPM along with Node.js.
Step 2: Verify Installation
To ensure Node.js and NPM are installed correctly:
- Open your command prompt or terminal.
- Type the following commands:
node -v
This should return the version of Node.js you installed.
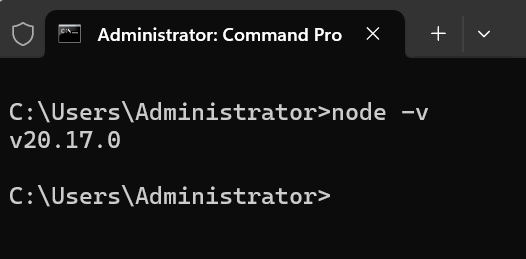
Step 3: Update NPM (Optional)
Occasionally, the version of NPM installed with Node.js might not be the latest. To update:
npm install -g npm@latest
This command will update NPM to its latest version globally on your system.
5. Creating Your First React App
The easiest way to set up a new React project is by using Create React App. This command-line tool provides an optimized and ready-to-go setup for React projects.
Step 1: Install Create React App Globally
If it’s your first time setting up a React environment, install create-react-app
globally:
npm install -g create-react-app
Step 2: Create a New React App
Once installed, you can create a new React project:
npx create-react-app my-first-react-app
Replace my-first-react-app
with your preferred project name. The npx
command runs the create-react-app
without needing to install it globally each time.
Step 3: Start Your React Application
To run the app:
- Navigate to your project directory:
cd my-first-react-app
2. Start the development server:
npm start
3. Open your browser and go to http://localhost:3000
to see your new React app.
6. Exploring the Folder Structure of a React App
Here’s a quick breakdown of the main folders in your React app:
- node_modules: Contains all the dependencies your project needs. Managed by NPM.
- public: Stores static files (e.g.,
index.html
, images) and can be directly accessed. - src: Contains your JavaScript, CSS, and other files where you’ll build your app.
- App.js: The main React component where your application starts.
- index.js: Entry point of your app where
App.js
is rendered.
Note: Avoid modifying files in
node_modules
. Instead, make all changes in thesrc
folder.
7. Best Practices for a React Environment
To set up an optimal React development environment, here are some best practices to follow:
1. Use Environment Variables
Manage sensitive data (like API keys) using environment variables. Create a .env
file in your root directory and store variables like this:
REACT_APP_API_URL=https://api.example.com
Important: Prefix React environment variables with
REACT_APP_
.
2. Organize Components
Create folders for different types of components (e.g., components
, pages
) to maintain a clean structure. Group similar files together.
3. Use ESLint and Prettier
Install ESLint for linting (checking for errors) and Prettier for code formatting:
npm install eslint prettier --save-dev
They help maintain clean and readable code.
4. Use Version Control
Track code changes using Git and repositories like GitHub or GitLab. This is especially useful for collaborative projects.
5. Regularly Update Dependencies
Check for dependency updates to ensure security and compatibility:
npm outdated
npm update
Pro Tip: Use tools like
npm-check
for a more interactive update experience.
FAQs
1. What is the role of Node.js in React?
Node.js provides a runtime environment for JavaScript, allowing you to install NPM packages and use tools like Create React App, which simplifies project setup.
2. Why use Create React App?
Create React App configures an optimized environment automatically, helping beginners avoid setup complexities while offering a ready-to-use structure for experienced developers.
3. Can I use React without NPM?
Yes, it’s possible to add React via CDN links directly in HTML files, but this approach lacks package management benefits and is less practical for large applications.
4. How often should I update dependencies?
Regular updates are recommended, especially for security patches. Use tools like Dependabot (GitHub) to automate dependency checks.
5. How can I deploy my React app?
After developing, you can deploy using services like Vercel, Netlify, or GitHub Pages. Run npm run build
to create an optimized production build.
Setting up a React development environment may seem challenging, but with Node.js and NPM, it’s straightforward. By following these steps and best practices, you can streamline your React setup and focus on building high-quality applications!
Thank you for reading! If you found this guide helpful and want to stay updated on more React.js content, be sure to follow us for the latest tutorials and insights: JavaDZone React.js Tutorials. Happy coding!