Introduction to Spring Boot Events
In this comprehensive guide, we will explore the world of event-driven programming in Spring Boot. Spring Boot events-driven architecture offers modularity, asynchronous communication, and loose coupling. We’ll cover event concepts, real-time examples, and demonstrate how to run a Spring Boot application with custom event listeners to harness the power of Spring Boot events.
Table of Contents
Understanding Event-Driven Programming
Event-driven programming is a powerful paradigm with these key aspects:
1. Loose Coupling and Modularity: Components are decoupled and don’t directly reference each other.
2. Asynchronous Communication: Components communicate by emitting and listening to events, enabling parallel execution.
Key Actors:
- Source: Publishes events, initiating actions.
- Event: Carries data and context about the source.
- Event Handler: Processes specific event types.
- Event Listener: Listens for events, identifies handlers, and delegates events for processing.
Real-Time Example:
Imagine a financial application notifying users of transactions via SMS and WhatsApp. We’ll model this with Spring Boot.
class TransactionNotificationEvent extends ApplicationEvent {
private String mobileNo;
private String accountNo;
private String operationType;
private double operatingAmount;
private double balance;
private String atmMachineNo;
public TransactionNotificationEvent(Object source) {
super(source);
}
// Accessors
}
Meet the TransactionNotificationEvent—an essential player in our financial application. Its job is simple but crucial: to hold all the vital details of a financial transaction. Imagine it as a data-packed envelope, carrying information like mobile numbers, account specifics, and transaction types. Whenever a customer initiates a transaction, this event springs to life, ready to trigger a series of actions in our event-driven system.
class WhatsAppNotificationEventListener implements ApplicationListener<TransactionNotificationEvent> {
public void onApplicationEvent(TransactionNotificationEvent event) {
// Read data from the event and send a WhatsApp message to the customer.
}
}
Introducing the WhatsAppNotificationEventListener—an attentive messenger in our system. Its mission is specific: to ensure customers receive prompt WhatsApp notifications about their transactions. Think of it as the guardian on the lookout for one event—TransactionNotificationEvent. When this event happens, it instantly acts, simulating the process of sending a WhatsApp notification to the customer. This class illustrates how events turn into real-world actions.
class AtmMachine implements ApplicationEventPublisherAware {
private ApplicationEventPublisher applicationEventPublisher;
public String withdrawal(String accountNo, double amount) {
// Logic to verify balance, deduct amount, and update the database
TransactionNotificationEvent event = new TransactionNotificationEvent(this);
event.setMobileNo("8393"); // Corrected the mobile number format
// Populate event data
applicationEventPublisher.publishEvent(event);
}
void setApplicationEventPublisher(ApplicationEventPublisher applicationEventPublisher) {
this.applicationEventPublisher = applicationEventPublisher;
}
}
And now, let’s meet our digital ATM—AtmMachine. This class embodies the heart of our system. It not only initiates transactions but also ensures their success. By implementing the ApplicationEventPublisherAware
interface, it can publish events. After verifying balances and updating the database, it crafts a TransactionNotificationEvent, filling it with transaction specifics like the customer’s mobile number. Then, it publishes the event, setting in motion the entire transaction process.
How to Work with Event-Driven Programming in Spring Framework?
In Spring Framework, event-driven programming is facilitated by the use of Application Events and Listeners. Here’s a simplified example:
AnEvent.java:
Step-1: Creating Spring Boot Events
Create Your Event Class: The journey begins with the creation of the AnEvent class, a crucial step in understanding Spring Boot Events. Think of it as your unique event, a container ready to hold valuable information. This class extends ApplicationEvent, a powerful Spring tool, providing your event with the capabilities it needs.
class AnEvent extends ApplicationEvent {
// Data to be passed to the handler as part of this event
public AnEvent(Object source) {
super(source);
}
}
Why We Need It: Explore the core purpose behind creating the AnEvent class. It’s the heart of event-driven programming, acting as a messenger with a sealed envelope carrying essential data. Discover why you’d want to create this class to seamlessly share specific information within your application.
Step-2: Spring Boot Event Listeners
The Event’s Trusty Guardian: Get to know the AnEventListener, a vital character in the Spring Boot Events storyline. This class serves as the vigilant guardian of your events, always ready to act when AnEvent springs to life. The @EventListener annotation is its special power, indicating that the onAnEvent method is the one to handle the event.
class AnEventSource {
@Autowired
private ApplicationEventPublisher publisher;
public void action() {
AnEvent event = new AnEvent(this);
publisher.publishEvent(event);
}
}
Why It Matters: Imagine your application as a grand narrative, and the AnEventListener is a character awaiting a pivotal moment. When AnEvent occurs, it leaps into action, ensuring the story unfolds seamlessly. Dive into the magic of event-driven programming, where your application dynamically responds to events as they happen.
Spring Boot Application Events & Listeners
During the startup of a Spring Boot application, various activities and stages occur, such as configuring the environment and initializing the IoC container. Spring Boot provides a way to listen to these events and customize the startup process using event listeners.
Types of Events Published by SpringApplication class:
- ApplicationStartingEvent: Published before any operation begins when calling
SpringApplication.run()
. - ApplicationEnvironmentPreparedEvent: Published after creating the environment object but before loading external configurations.
- ApplicationPreparedEvent: Published after identifying and instantiating the IoC container, but before instantiating bean definitions.
- ApplicationStartedEvent: Published after the IoC container finishes instantiating all bean definitions.
- ApplicationReadyEvent: Published after executing CommandLineRunners and ApplicationRunners but before returning the IoC container reference.
- ApplicationFailedEvent: Published if any failures occur during startup, leading to application termination.
Creating Custom Spring Boot Events
Here’s how you can create a custom listener to handle a specific event during Spring Boot application startup:
class MyApplicationStartedEventListener {
@EventListener
public void onApplicationStartedEvent(ApplicationStartedEvent event) {
// Custom logic to execute when the application starts
}
}
@SpringBootApplication
class EventApplication {
public static void main(String[] args) {
MyApplicationStartedEventListener listener = new MyApplicationStartedEventListener();
SpringApplication springApplication = new SpringApplicationBuilder(EventApplication.class)
.listeners(listener).build();
ApplicationContext context = springApplication.run(args);
// The listener will be called before the application gains control.
}
}
Additional Spring Boot Events Examples
1. Creating Additional Custom Events
// Custom event class
class CustomEvent extends ApplicationEvent {
public CustomEvent(Object source) {
super(source);
}
}
// Custom event listener
class CustomEventListener {
@EventListener
public void onCustomEvent(CustomEvent event) {
// Handle the custom event
System.out.println("Custom event handled.");
}
}
2. Spring Boot Asynchronous Event Example:
// Asynchronous event class
class AsynchronousEvent extends ApplicationEvent {
public AsynchronousEvent(Object source) {
super(source);
}
}
// Asynchronous event listener
class AsynchronousEventListener {
@Async // Enable asynchronous processing
@EventListener
public void onAsynchronousEvent(AsynchronousEvent event) {
// Handle the asynchronous event asynchronously
System.out.println("Asynchronous event handled asynchronously.");
}
}
Spring Boot Events: A Brief Overview(Best Practices)
In Spring Boot, events are a powerful mechanism that allows different parts of your application to communicate asynchronously. Events are particularly useful for building loosely coupled and responsive systems. Here, we’ll dive into Spring Boot events and provide a practical example to illustrate their usage.
Creating a Custom Spring Boot Event
Imagine you’re building an e-commerce platform, and you want to send a notification to users whenever a new product is added to your catalog. Spring Boot events can help with this.
Step 1: Define the Event
import org.springframework.context.ApplicationEvent;
public class ProductAddedEvent extends ApplicationEvent {
private final String productName;
public ProductAddedEvent(Object source, String productName) {
super(source);
this.productName = productName;
}
public String getProductName() {
return productName;
}
}
Here, we’ve defined a custom event class, ProductAddedEvent
, which extends ApplicationEvent
. It carries information about the new product that was added.
Step 2: Create an Event Publisher
Next, we need a component that will publish this event when a new product is added. For instance, we can create a service called ProductService
:
import org.springframework.context.ApplicationEventPublisher;
import org.springframework.stereotype.Service;
@Service
public class ProductService {
private final ApplicationEventPublisher eventPublisher;
public ProductService(ApplicationEventPublisher eventPublisher) {
this.eventPublisher = eventPublisher;
}
public void addProduct(String productName) {
// Add the product to the catalog
// ...
// Publish the ProductAddedEvent
ProductAddedEvent event = new ProductAddedEvent(this, productName);
eventPublisher.publishEvent(event);
}
}
In the addProduct
method, we first add the new product to the catalog (this is where your business logic would go), and then we publish the ProductAddedEvent
. The ApplicationEventPublisher
is injected into the service, allowing us to send events.
Step 3: Create an Event Listener
Now, let’s create a listener that will respond to the ProductAddedEvent
by sending notifications to users. In this example, we’ll simulate sending emails:
import org.springframework.context.event.EventListener;
import org.springframework.stereotype.Component;
@Component
public class EmailNotificationListener {
@EventListener
public void handleProductAddedEvent(ProductAddedEvent event) {
// Get the product name from the event
String productName = event.getProductName();
// Send an email notification to users
// ...
System.out.println("Sent email notification for product: " + productName);
}
}
This listener is annotated with @Component
to make it a Spring-managed bean. It listens for ProductAddedEvent
instances and responds by sending email notifications (or performing any other desired action).
Best Practices for Spring Boot Events
Now that we’ve seen an example of Spring Boot events in action, let’s explore some best practices:
- Use Events for Decoupling: Events allow you to decouple different parts of your application. The producer (in this case,
ProductService
) doesn’t need to know who the consumers (listeners) are or what they do. This promotes modularity and flexibility. - Keep Events Simple: Events should carry only essential information. Avoid making them too complex. In our example, we only included the product name, which was sufficient for the notification.
- Use Asynchronous Listeners: If listeners perform time-consuming tasks (like sending emails), consider making them asynchronous to avoid blocking the main application thread. You can use the
@Async
annotation for this purpose. - Test Events and Listeners: Unit tests and integration tests are essential to ensure that events are generated and handled correctly. Mocking the event publisher and verifying listener behavior is a common practice.
- Document Events: Document your custom events and their intended usage. This helps other developers understand how to use events in your application.
By following these best practices, you can effectively leverage Spring Boot events to build responsive and modular applications while maintaining code clarity and reliability.
Running the Application:
- Create a new Spring Boot project or use an existing one.
- Copy and paste the provided code examples into the respective classes.
- Ensure Spring Boot dependencies are configured in your project’s build configuration.
- Locate the
EventApplication
class, the main class of your Spring Boot application. - Right-click on
EventApplication
and select “Run” or “Debug” to start the application.
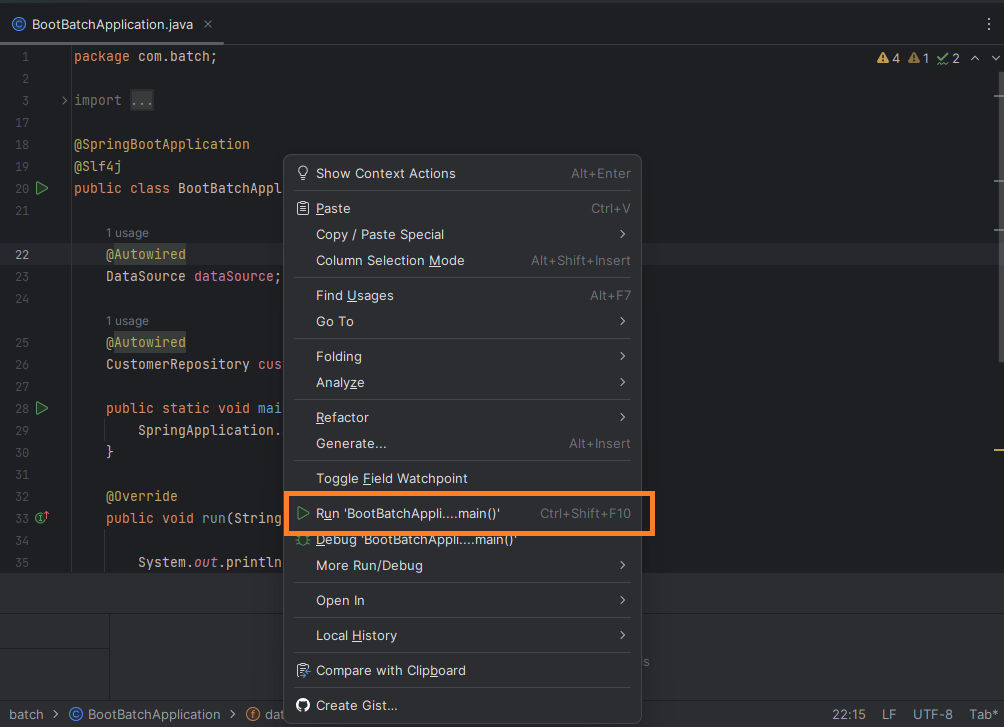
Observing Output:
- The custom logic within
MyApplicationStartedEventListener
will execute during application startup and print to the console. - Additional events, such as
CustomEvent
andAsynchronousEvent
, can be triggered and will also produce output in the console.
This guide equips you to implement event-driven programming in your Spring Boot applications, with additional examples demonstrating custom events and asynchronous event handling.
Related Articles:
This is what I never know was needed. Thank you
Well written with good examples and anyone can understand the logic quickly.
Thanks for the woderful post.
Thank you!!