In the world of microservices architecture, the way we manage data is critical. One of the most common mistakes developers make is over-relying on relational databases for every microservice, even when it’s not the best fit. Stop Using Relational Databases in Microservices: Polyglot Persistence Wins is the new mantra for building scalable and efficient systems. In this article, we’ll explore why polyglot persistence is the better approach and how it can vastly improve the performance and scalability of microservices.
What is Polyglot Persistence?
Before diving into the specifics, it’s essential to understand polyglot persistence. In simple terms, polyglot persistence is the practice of using different types of databases depending on the specific requirements of each microservice.
Instead of using a one-size-fits-all approach with relational databases, polyglot persistence encourages the use of the right database for the right job. This means you might use NoSQL databases (like MongoDB or Cassandra) for some services, graph databases (like Neo4j) for others, and relational databases (like MySQL or PostgreSQL) where they shine.
This approach ensures that your microservices are optimized, scalable, and efficient.
Bad Code: Over-Reliance on Relational Databases
Here’s an example of bad code where a relational database is used for every microservice, regardless of the need. This approach might work for small-scale systems but is prone to performance issues and scalability bottlenecks as your application grows.
public class ProductService {
@Autowired
private ProductRepository productRepository;
public Product getProductById(Long id) {
return productRepository.findById(id);
}
}
Why is this bad code?
- Limited scalability: Relational databases can be hard to scale horizontally when your data grows.
- Performance bottlenecks: For services requiring high throughput or low-latency data access, relational databases may not be efficient.
- Single point of failure: Relying on a single database increases the risk of service outages if the database fails.
Example Scenarios:
- Transactional data (such as banking systems) is well-suited for relational databases, but real-time data processing and high-volume reads, as seen in services like e-commerce or big data applications, may suffer performance issues when using relational databases alone.
Good Code: Polyglot Persistence in Microservices
Instead of relying on a single type of database, the polyglot persistence approach recommends using the best database for each microservice based on its specific requirements. By combining different database types, you can optimize your services for speed, reliability, and scalability.
Here’s an example of good code that uses polyglot persistence in a microservices environment:
public class ProductService {
@Autowired
private ProductRepository productRepository;
@Autowired
private NoSQLProductRepository noSQLProductRepository;
public Product getProductById(Long id) {
if (id < 1000) {
// Relational DB for small data
return productRepository.findById(id);
} else {
// NoSQL DB for high read throughput
return noSQLProductRepository.findById(id);
}
}
}
Why is this good code?
- Relational Database: It’s used for structured data and small datasets, leveraging the power of ACID transactions and complex queries.
- NoSQL Database: It’s used for high-volume, unstructured data where scalability and high throughput are essential, like in e-commerce systems where fast reads are crucial.
This method ensures that each service is optimized for its specific needs, leading to better performance and scalability.
Benefits of Polyglot Persistence in Microservices
By adopting polyglot persistence, microservices can achieve several key advantages:
- Optimized performance: Use the best database suited to each service’s data model and access patterns.
- Scalability: Horizontal scaling is possible with NoSQL databases, enabling you to easily scale as traffic and data grow.
- Flexibility: Choose the right database for the task – whether it’s a relational, NoSQL, or graph database.
- Fault tolerance: If one database goes down, others can continue to function without major disruptions to the system.
Key Differences Between Relational Databases, NoSQL, and Graph Databases
To help you better understand when to use each type of database, we’ve outlined the key differences in the table below.
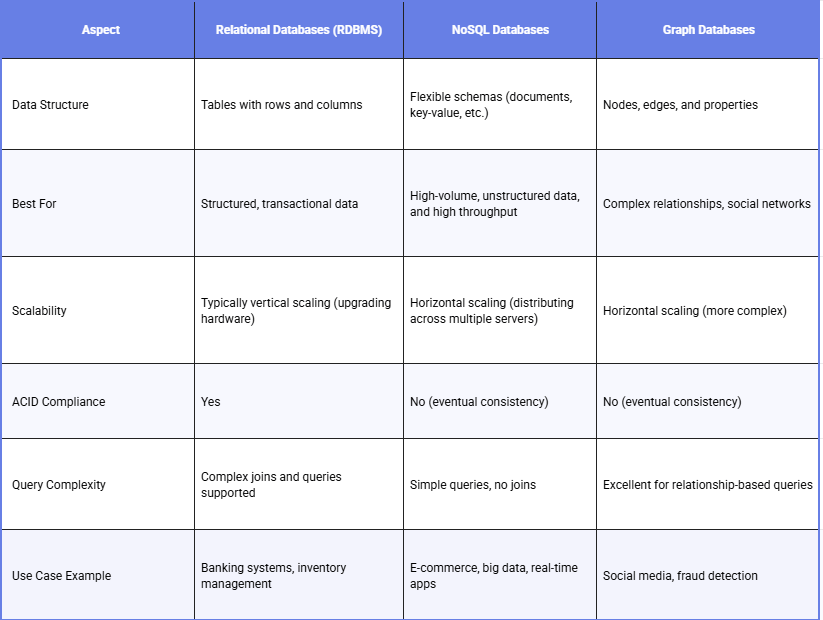
Real-World Examples of Polyglot Persistence
Let’s now look at some real-world scenarios where polyglot persistence shines:
Example 1: Content Management System (CMS)
In a CMS for a news website, the platform needs to handle structured data (like users and articles) and unstructured data (like comments and media files).
- Relational Database (PostgreSQL): Store user profiles and metadata about articles.
- NoSQL Database (MongoDB): Store article content and user comments, which can vary in format.
- Object Storage (Amazon S3): Store large media files like images and videos.
public class ArticleService {
@Autowired
private ArticleRepository articleRepository;
public List<Article> getArticlesByAuthor(String author) {
return articleRepository.findByAuthor(author);
}
}
Example 2: E-Commerce Platform
For an e-commerce platform, managing transactions and product catalogs requires different approaches.
- Relational Database (PostgreSQL): Store transactional data like orders and inventory.
- NoSQL Database (MongoDB): Store product catalog and user reviews.
- Cache (Redis): Cache shopping cart data to improve performance.
public class CartService {
@Autowired
private RedisCartRepository redisCartRepository;
public Cart getCartForUser(String userId) {
return redisCartRepository.get(userId);
}
}
Example 3: Social Media Platform
In a social media platform, the need to manage relationships, posts, and comments calls for the use of different database types.
- Relational Database (PostgreSQL): Store user profiles and relationships.
- NoSQL Database (MongoDB): Store posts and comments.
- Graph Database (Neo4j): Store and query user relationships, such as followers and friends.
public class SocialService {
@Autowired
private GraphUserRepository graphUserRepository;
public List<User> getFollowers(String username) {
return graphUserRepository.findFollowers(username);
}
}
Conclusion: Stop Using Relational Databases for Microservices: Polyglot Persistence Wins
In conclusion, polyglot persistence is the key to unlocking the full potential of microservices architecture. Instead of forcing all services to use a single type of database, adopting the right database for each microservice will result in better performance, scalability, and flexibility.
So, the next time you’re designing a system, don’t just settle for relational databases. Stop using relational databases for microservices where they don’t fit and let polyglot persistence lead the way to more efficient and scalable solutions.
Key Takeaways:
- Don’t rely on just one type of database for all services.
- Polyglot persistence allows you to use the right database for the right task.
- It improves performance, scalability, and fault tolerance across services.
By embracing polyglot persistence, you are setting your microservices architecture up for success!