In the world of Microservices architecture, efficiently managing configurations across multiple services is crucial. “Reload Application Properties in Spring Boot” becomes even more significant when it comes to updating configurations and ensuring synchronization, as well as refreshing config changes. However, with the right tools and practices, like Spring Cloud Config and Spring Boot Actuator, this process can be streamlined. In this guide, we’ll delve into how to effectively propagate updated configurations to all Config Clients (Microservices) while maintaining synchronization.
Table of Contents
Spring Cloud Config Server Auto-Reload
When you make changes to a configuration file in your config repository and commit those changes, the Spring Cloud Config Server, configured to automatically reload the updated configurations, becomes incredibly useful for keeping your microservices up to date.
To set up auto-reloading, you need to configure the refresh rate in the Config Server’s configuration file, typically located in application.yml or application.properties. The “Reload Application Properties in Spring Boot” guide will walk you through this process, with a focus on the refresh-rate property, specifying how often the Config Server checks for updates and reloads configurations.
spring:
cloud:
config:
server:
git:
refresh-rate: 3000 # Set the refresh rate in milliseconds
Refresh Config Clients with Spring Boot Actuator
To get started, add the Spring Boot Actuator dependency to your microservice’s project. You can do this by adding the following lines to your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
While the Config Server reloads configurations automatically, the updated settings are not automatically pushed to the Config Clients (microservices). To make sure these changes are reflected in the Config Clients, you must trigger a refresh.
This is where the “Reload Application Properties in Spring Boot” guide becomes crucial. Spring Boot Actuator provides various management endpoints, including the refresh endpoint, which is essential for updating configurations in Config Clients.
Reload Application Properties in Spring Boot: Exposing the Refresh Endpoint
Next, you need to configure Actuator to expose the refresh endpoint. This can be done in your microservice’s application.yml
or .properties
file:
management:
endpoint:
refresh:
enabled: true
endpoints:
web:
exposure:
include: refresh
Java Code Example
Below is a Java code example that demonstrates how to trigger configuration refresh in a Config Client microservice using Spring Boot:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.context.config.annotation.RefreshScope;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.cloud.context.refresh.ContextRefresher;
@SpringBootApplication
public class ConfigClientApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigClientApplication.class, args);
}
}
@RestController
@RefreshScope
public class MyController {
@Value("${example.property}")
private String exampleProperty;
private final ContextRefresher contextRefresher;
public MyController(ContextRefresher contextRefresher) {
this.contextRefresher = contextRefresher;
}
@GetMapping("/example")
public String getExampleProperty() {
return exampleProperty;
}
@GetMapping("/refresh")
public String refresh() {
contextRefresher.refresh();
return "Configuration Refreshed!";
}
}
- Reload Application Properties:
- To trigger a refresh in a Config Client microservice, initiate a POST request to the refresh endpoint. For example:
http://localhost:8080/actuator/refresh
. - This request will generate a refresh event within the microservice.
Send a POST Request with Postman Now, open Postman and create a POST request to your microservice’s refresh endpoint. The URL should look something like this:
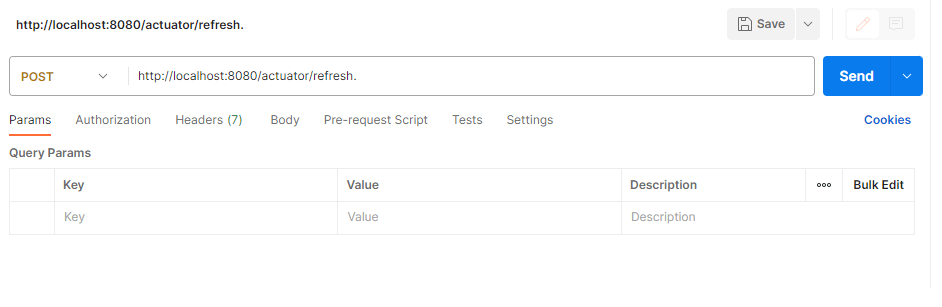
2. Bean Reloading:
- Configurations injected via
@Value
annotations in bean definitions adorned with@RefreshScope
will be reloaded when a refresh is triggered. - If values are injected through
@ConfigurationProperties
, the IOC container will automatically reload the configuration.
By following these steps and incorporating the provided Java code example, you can effectively ensure that updated configurations are propagated to your Config Clients, and their synchronization is managed seamlessly using Spring Cloud Config and Spring Boot Actuator. This approach streamlines configuration management in your microservices architecture, allowing you to keep your services up to date efficiently and hassle-free.
“In this guide, we’ve explored the intricacies of Spring Cloud Config and Spring Boot Actuator in efficiently managing and refreshing configuration changes in your microservices architecture. To delve deeper into these tools and practices, you can learn more about Spring Cloud Config and its capabilities. By leveraging these technologies, you can enhance your configuration management and synchronization, ensuring seamless operations across your microservices.”
Related Articles: